กราฟ¶
ทบทวน¶
Vector¶
แหล่งอ้างอิง: https://www.cplusplus.com/reference/vector/vector/
1#include <bits/stdc++.h>
2using namespace std;
3int main() {
4 int n, x;
5 vector<int> a;
6 cin >> n;
7 for (auto i=0; i<n; i++) {
8 cin >> x;
9 a.push_back(x);
10 }
11 a.erase(a.begin()+3, a.begin()+5);
12 for (auto t=a.begin(); t!=a.end(); t++)
13 cout << *t << endl;
14 return 0;
15}
Queue¶
แหล่งอ้างอิง: https://www.cplusplus.com/reference/queue/queue/
1#include <bits/stdc++.h>
2using namespace std;
3int main() {
4 int n, q;
5 queue<string> names;
6 string name;
7 cin >> n;
8 for (int i=0; i<n; i++) {
9 cin >> name;
10 names.push(name);
11 }
12 while (!names.empty()) {
13 cout << names.front() << endl;
14 names.pop();
15 }
16 return 0;
17}
Priority Queue¶
แหล่งอ้างอิง: https://www.cplusplus.com/reference/queue/priority_queue/
1#include <bits/stdc++.h>
2using namespace std;
3int main() {
4 priority_queue<int> a;
5 int n, x;
6 cin >> n;
7 for (int i=0; i<n; i++) {
8 cin >> x;
9 a.push(x);
10 }
11 cout << "max = " << a.top() << endl;
12 return 0;
13}
ความหมาย¶
กราฟเป็นโครงสร้างข้อมูลที่มีข้อมูลของตำแหน่ง (Vertex) และข้อมูลความสัมพันธ์ระหว่างตำแหน่ง (Edge)
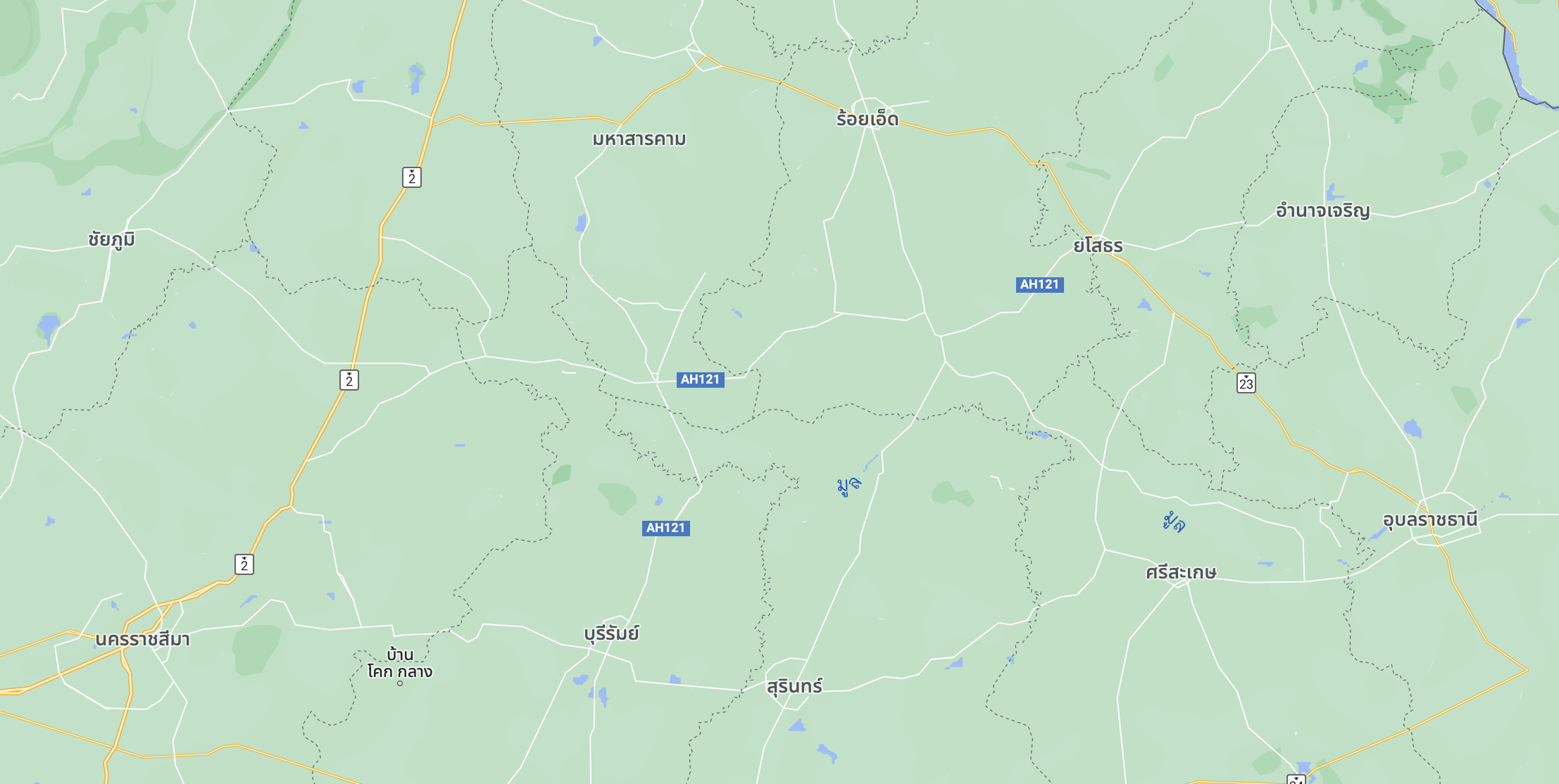
ตัวอย่างการใช้กราฟเพื่อแทนตำแหน่งและระยะห่างระหว่างจังหวัด
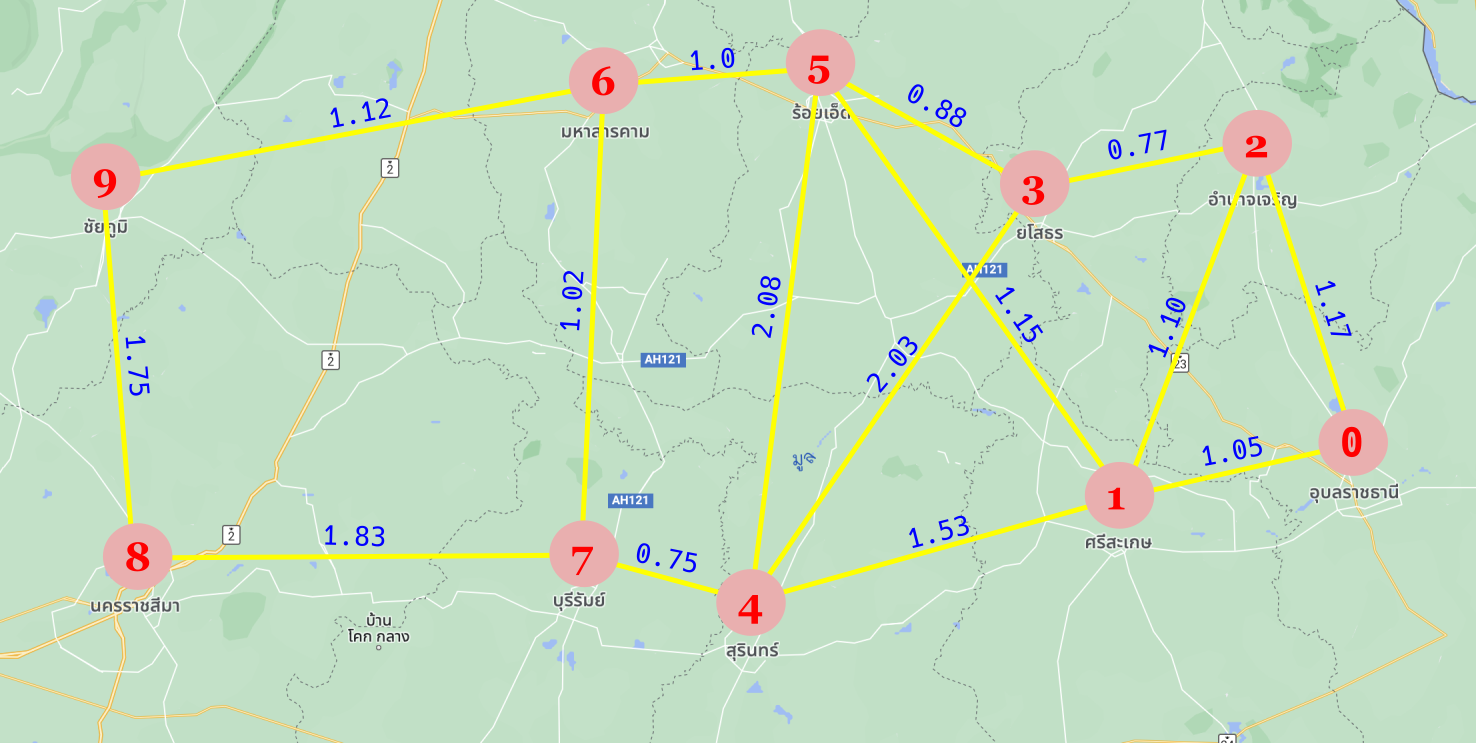